Orbital Scoreboard
VPX Sample Integration
4min
VisualBasic
1'XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
2' X X X X X X X X X X X X X X X X X X X X X X X
3'/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/ \/
4' Orbital Scoreboard Code
5'\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\ /\
6' X X X X X X X X X X X X X X X X X X X X X X X
7
8 '****************************
9 ' POST SCORES
10 '****************************
11 Dim osbtemp:osbtemp = osbdefinit
12 Dim osbtempscore:osbtempscore = 0
13 Sub SubmitOSBScore
14 On Error Resume Next
15 if osbactive = 1 or osbactive = 2 Then
16 Dim objXmlHttpMain, Url, strJSONToSend
17
18 Url = "https://hook.integromat.com/82bu988v9grj31vxjklh2e4s6h97rnu0"
19
20 strJSONToSend = "{""auth"":""" & osbkey &""",""player id"": """ & osbid & """,""player initials"": """ & osbtemp &""",""score"": " & CStr(osbtempscore) & ",""table"":"""& TableName & """,""version"":""" & myVersion & """}"
21
22 Set objXmlHttpMain = CreateObject("Msxml2.ServerXMLHTTP")
23 objXmlHttpMain.open "PUT",Url, False
24 objXmlHttpMain.setRequestHeader "Content-Type", "application/json"
25 objXmlHttpMain.setRequestHeader "application", "application/json"
26
27 objXmlHttpMain.send strJSONToSend
28 end if
29 End Sub
30
31
32 '****************************
33 ' GET SCORES
34 '****************************
35 dim worldscores
36
37 Sub GetScores()
38 if osbkey="" then exit sub
39 On Error Resume Next
40 Dim objXmlHttpMain, Url, strJSONToSend
41
42 Url = "https://hook.integromat.com/kj765ojs42ac3w4915elqj5b870jrm5c"
43
44 strJSONToSend = "{""auth"":"""& osbkey &""", ""table"":"""& TableName & """}"
45
46 Set objXmlHttpMain = CreateObject("Msxml2.ServerXMLHTTP")
47 objXmlHttpMain.open "PUT",Url, False
48 objXmlHttpMain.setRequestHeader "Content-Type", "application/json"
49 objXmlHttpMain.setRequestHeader "application", "application/json"
50
51 objXmlHttpMain.send strJSONToSend
52
53 worldscores = objXmlHttpMain.responseText
54 vpmtimer.addtimer 3000, "showsuccess '"
55 debug.print "got the scores"
56 'debug.print worldscores
57 splitscores
58 End Sub
59
60 Dim scorevar(22)
61 Dim dailyvar(22)
62 Dim weeklyvar(22)
63 Dim alltimevar(42)
64 sub emptyscores
65 dim i
66 For i = 0 to 42
67 alltimevar(i) = "0"
68 Next
69 For i = 0 to 22
70 weeklyvar(i) = "0"
71 dailyvar(i) = "0"
72 Next
73 End Sub
74 emptyscores
75
76
77 Sub splitscores
78 On Error Resume Next
79 dim a,scoreset,subset,subit,myNum,daily,weekly,alltime,x
80 a = Split(worldscores,": {")
81 subset = Split(a(1),"[")
82
83' debug.print subset(1)
84' debug.print subset(2)
85' debug.print subset(3)
86' daily scores
87 myNum = 0
88 daily = Split(subset(1),": ")
89 for each x in daily
90 myNum = MyNum + 1
91 x = Replace(x, vbCr, "")
92 x = Replace(x, vbLf, "")
93 x = Replace(x, ",", "")
94 x = Replace(x, """", "")
95 x = Replace(x, "{", "")
96 x = Replace(x, "}", "")
97 x = Replace(x, "score", "")
98 x = Replace(x, "initials", "")
99 x = Replace(x, "weekly", "")
100 x = Replace(x, "]", "")
101 x = Replace(x, "alltime", "")
102 dailyvar(MyNum) = x
103 if dailyvar(MyNum) = "" Then
104 if MyNum = 2 or 4 or 6 or 8 or 10 or 12 or 14 or 16 or 18 or 20 Then
105 dailyvar(MyNum) = "OBS"
106 Else
107 dailyvar(MyNum) = 0
108 end if
109 end if
110 debug.print "dailyvar(" &MyNum & ")=" & x
111 Next
112
113' weekly scores
114 myNum = 0
115 weekly = Split(subset(2),": ")
116 for each x in weekly
117 myNum = MyNum + 1
118 x = Replace(x, vbCr, "")
119 x = Replace(x, vbLf, "")
120 x = Replace(x, ",", "")
121 x = Replace(x, """", "")
122 x = Replace(x, "{", "")
123 x = Replace(x, "}", "")
124 x = Replace(x, "score", "")
125 x = Replace(x, "initials", "")
126 x = Replace(x, "weekly", "")
127 x = Replace(x, "]", "")
128 x = Replace(x, "alltime", "")
129 weeklyvar(MyNum) = x
130 if weeklyvar(MyNum) = "" Then
131 if MyNum = 2 or 4 or 6 or 8 or 10 or 12 or 14 or 16 or 18 or 20 Then
132 weeklyvar(MyNum) = "OBS"
133 Else
134 weeklyvar(MyNum) = 0
135 end if
136 end if
137 debug.print "weeklyvar(" &MyNum & ")=" & x
138 Next
139
140' alltime scores
141 myNum = 0
142 alltime = Split(subset(3),": ")
143 for each x in alltime
144 myNum = MyNum + 1
145 x = Replace(x, vbCr, "")
146 x = Replace(x, vbLf, "")
147 x = Replace(x, ",", "")
148 x = Replace(x, """", "")
149 x = Replace(x, "{", "")
150 x = Replace(x, "}", "")
151 x = Replace(x, "score", "")
152 x = Replace(x, "initials", "")
153 x = Replace(x, "weekly", "")
154 x = Replace(x, "]", "")
155 x = Replace(x, "alltime", "")
156 alltimevar(MyNum) = x
157 if alltimevar(MyNum) = "" Then
158 if MyNum = 2 or 4 or 6 or 8 or 10 or 12 or 14 or 16 or 18 or 20 or 22 or 24 or 26 or 28 or 30 or 32 or 34 or 36 or 38 or 40 Then
159 alltimevar(MyNum) = "OBS"
160 Else
161 alltimevar(MyNum) = "0"
162 end if
163 end if
164 debug.print "alltimevar(" &MyNum & ")=" & x
165 Next
166
167 end Sub
168
169
170 sub showsuccess
171 pNote "Scoreboard","Updated"
172 'pupDMDDisplay "-","Scoreboard^Updated",dmdnote,3,0,10
173 end sub
174
175 GetScores
VisualBasic
1 Sub CheckHighscore()
2 Dim tmp
3 tmp = Score(CurrentPlayer)
4 osbtempscore = Score(CurrentPlayer)
5
6 If tmp > HighScore(3) Then
7 AwardSpecial
8 vpmtimer.addtimer 2000, "PlaySound ""vo_contratulationsgreatscore"" '"
9 HighScore(3) = tmp
10 'enter player's name
11 HighScoreEntryInit()
12 DOF 403, DOFPulse 'DOF MX - Hi Score
13 Else
14 osbtemp = osbdefinit
15 SubmitOSBScore
16 EndOfBallComplete
17 End If
18 End Sub
VisualBasic
1 Sub HighScoreCommitName()
2 PuPlayer.playresume 4
3 playclear pBackglass
4 PuPlayer.SetLoop 2,0
5 PuPlayer.playresume 4
6 playclear pAudio
7 PuPlayer.SetLoop 7,0
8 hsEnteredName = hsEnteredDigits(1) & hsEnteredDigits(2) & hsEnteredDigits(3)
9 HighScoreName(3) = hsEnteredName
10 checkorder
11 osbtemp = hsEnteredName
12 SubmitOSBScore
13 EndOfBallComplete()
14 PuPlayer.LabelSet pBackglass,"HighScore","",1,""
15 PuPlayer.LabelSet pBackglass,"HighScoreL1","",1,""
16 PuPlayer.LabelSet pBackglass,"HighScoreL2"," ",1,""
17 PuPlayer.LabelSet pBackglass,"HighScoreL3"," ",1,""
18 PuPlayer.LabelSet pBackglass,"HighScoreL4"," ",1,""
19 hsbModeActive = False
20 End Sub
Updated 25 Mar 2024
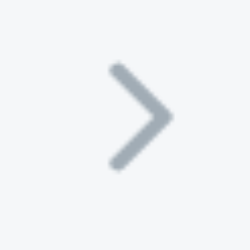
Did this page help you?